FFmpeg is a powerful multimedia framework used for processing audio and video files. AC3(Audio Codec 3) is a widely adopted audio codec known for its surround sound capabilities.Typically, AC3 is a lossy codec, meaning it compresses audio by discarding some data. However,AC3PT, or AC3 Pass-Through, allows for the direct transmission of AC3 audio streams without reencoding,ensuring a lossless experience that preserves the original audio quality. This isparticularly important for applications requiring high fidelity. Additionally, AC3PT ensures compatibility with Dolby Digital systems, which can decode raw AC3 streams. In contrast, ifstandard decoders are used, the audio will be lossy, as they do not support this pass-throughmethod.
Integrating AC3PT into FFmpeg is essential for developers who want to maintain the original audio quality while efficiently handling AC3 streams in their workflows. This guide will cover the steps needed to implement AC3PT in FFmpeg. These steps also apply to the implementation of any new codec that is not natively supported by FFmpeg.
Need for AC3PT When You Have AC3
AC3PT (AC3 Pass-Through) is essential even when AC3 is available because it allows for the direct transmission of AC3 audio streams without re-encoding. This ensures the preservation of original audio quality, emphasising that the process is lossless. This is crucial for applications that demand high fidelity—often reaching up to 640 kbps for surround sound configurations.
Using AC3PT reduces processing overhead, leading to latency improvements; in some cases, it can decrease processing time by up to 50% compared to re-encoding. This is particularly valuable in real-time scenarios like live broadcasting or gaming, where every millisecond counts.
Additionally, AC3PT ensures compatibility with multi-channel audio systems, maintaining audio integrity for formats supporting up to 5.1 or 7.1 surround sound configurations. While AC3PT does not compress file sizes, it enables more efficient audio management in workflows, making it an important option for developers and media professionals.a
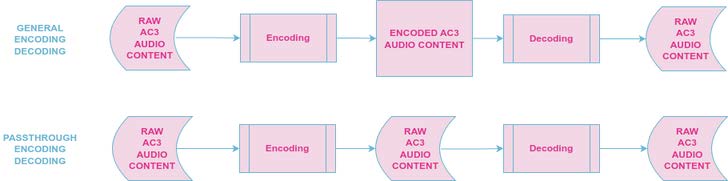
Integrating AC3PT in FFmpeg: Implementation Steps
To integrate AC3PT (AC3 Pass-Through) into FFmpeg, follow these implementation steps:
Preliminary Actions
Have a look at the AVCodec Structure in the ffmpeg.org/doxygen/trunk/structFFCodec.html, with this structure, we’ll get an idea on how to use this structure for AC3PT Implementation in FFmpeg.
Any new codec introduced should have it’s own encoder, decoder code implementation in libavcodec. It also should have it’s codec description in libavcodec/codec_desc.c, encoder and decoder entries in libavcodec/allcodecs.c and an entry in the libavcodec/Makefile.
We’ll begin with writing the encoder and the decoder for AC3PT inside the libavcodec.
Encoder File
To integrate AC3PT encoding into FFmpeg, begin by creating an encoder file. It is convention to name encoder files ending with enc. Hence we’ll use ac3ptenc.c.
Now start writing the file by including essential headers required such as avcodec.h, encode.h, etc. Next, define constants for the number of channels, bytes per sample, and specific AC3 values like the preamble and sync word.
Create an enumeration for parsing states to effectively manage the different stages of the audio processing workflow. Then, define a structure to hold the state information, sync frame size, position in the data burst, and buffers for aggregating audio data.
Implement the initialisation function to set the initial parsing state of the context. This prepares the codec for handling incoming audio frames.
Implement an encoding function. Inside it, develop a loop to process the audio buffer. Create a mechanism to handle different parsing states. For each state, check for key values such as the preamble and sync word, updating the parsing state accordingly. Manage memory for the aggregation buffer carefully, ensuring it is allocated and freed properly to avoid leaks.
Finally, define the FFCodec structure, specifying the codec name, type, ID, private data size, initialisation function, encoding callback, and relevant capabilities. Incorporate robust error handling, particularly for memory allocation and processing steps, to ensure stability and reliability in the codec’s operation. This structured approach will facilitate a successful integration of AC3PT encoding into FFmpeg.
Here is the code skeleton that you can use.
typedef enum
{
// States for parsing AC3PT frames
} ac3ptParseState;
typedef struct ac3ptEncContext
{
// For holding parsing state and context information
// Uses the private data from the AVCodecContext
} ac3ptEncContext;
static av_cold int ac3pt_encode_init(AVCodecContext *avctx)
{
// Initialize codec context and set initial state
}
static int ac3pt_encode_frame(AVCodecContext *avctx, AVPacket *avpkt,
const AVFrame *avframe, int *got_packet_ptr)
{
// Process audio frames and update output AVPacket
// Manage states and handle memory as needed
}
const FFCodec ff_ac3pt_encoder = {
.p.name = "ac3pt",
CODEC_LONG_NAME("AC3PT (AC3 Passthrough)"),
.p.type = AVMEDIA_TYPE_AUDIO,
.p.id = AV_CODEC_ID_AC3,
.priv_data_size = sizeof(ac3ptEncContext), // Size of context structure
.init = ac3pt_encode_init, // Initialization function pointer
FF_CODEC_ENCODE_CB(ac3pt_encode_frame), // Frame encoding callback
.p.capabilities = // Specify codec capabilities
};
Use libavcodec/ac3enc_fixed.c and libavcodec/ac3enc_float.c for reference.
Decoder File
Similar to AC3PT encoding, we begin by creating a decoder file. It is convention to name decoder
files ending with dec in FFmpeg. Hence we’ll use ac3ptdec.c.
Now start including the necessary headers that provide access to core functionalities and data structures for audio decoding. These headers should encompass standard libraries, codec internals, and specific parsers needed for AC3 audio streams.
In the initialisation function, initialise the codec context. Set the output to the desired audio format, ensuring it conforms to specifications such as sample rate and bit depth. Specifically, configure the sample format to the appropriate type, define the channel layout, and specify the sample rate.
The next critical function is to parse frame header. It is responsible for extracting metadata from the AC3 frame. Use ff_ac3_parse_header to populate an AC3HeaderInfo structure with relevant information. Validate key properties such as the bitstream ID and sample rate to ensure compatibility. Create mechanism to handle unsupported formats.
Following this, the write function should be implemented to fill the output frame buffers. Start by clearing the buffers to prevent residual data issues. Write a preamble header based on SMPTE standards, which requires careful handling of byte order and specific bit assignments.
Create a decode function to manage the overall decoding process. Begin by validating input parameters to avoid null pointer dereferences and ensure data integrity. Copy the input buffer to a temporary buffer, which will prevent any out-of-bounds access. Initialise the bitstream context with the starting point of the AC3 frame data.
Call parse_frame_header already created to extract frame metadata, handle any arising errors gracefully. After parsing, verify that the frame size is valid and does not exceed buffer limits to avoid overflow issues. Allocate memory for frame buffers based on the number of samples needed, which can be calculated using the sample rate.
Conclude the implementation by defining the FFcodec structure, which ties everything together. Populate the codec structure with necessary fields such as name, type, ID, and pointers to initialisation and decoding functions. This final step ensures that your codec can be recognised and properly utilised within the broader framework.
By adhering to these guidelines, the AC3 pass-through decoder will be structured, functional, and maintainable, allowing for easier understanding and potential future enhancements. Each section corresponds to critical components of the decoding process, facilitating a coherent and efficient implementation.
Here is the code skeleton that you can use.
static av_cold int _decode_init(AVCodecContext *avctx)
{
// Initialize codec context and set output format to 48 kHz, 16-bit PCM
}
static int _decode_frame(AVCodecContext* avctx, struct AVFrame *data, int*
got_frame_ptr, AVPacket* avpkt)
{
// Manage the decoding process for audio frames
// Validate input parameters to ensure data integrity
// Copy input buffer to a temporary buffer to prevent out-of-bounds
access
// Initialize bitstream context for AC3 frame data
// Call helper functions to parse frame headers and write data to the
output frame
}
const FFCodec ff__decoder = {
.p.name = "ac3pt",
CODEC_LONG_NAME("AC3PT (AC3 Passthrough)"),
.p.type = AVMEDIA_TYPE_AUDIO,
.p.id = AV_CODEC_ID_AC3,
.priv_data_size = sizeof(AC3DecoderContext), // Size of context struct
.init = _decode_init, // Initialization function pointer
FF_CODEC_DECODE_CB(_decode_frame), // Frame decoding callback
.p.capabilities = // Specify codec capabilities
};
Use ac3dec_fixed.c and ac3dec_float.c for reference.
Codec ID
Add your codec inside the codec_id.h::AVCodecID enum so that the new codec will be recognized by the FFmpeg. However, since AC3PT uses AV_CODEC_ID_AC3, we can omit this step and just remap AV_CODEC_ID_AC3 in libavcodec/codec_desc.c to AC3PT encoder/decoder by changing the name from ac3 to ac3pt.
…
{
.id = AV_CODEC_ID_AC3,
.type = AVMEDIA_TYPE_AUDIO,
.name = "ac3pt",
.long_name = NULL_IF_CONFIG_SMALL("AC3PT (AC3 Passthrough)"),
.props = AV_CODEC_PROP_LOSSLESS,
},
…
Entry for Encoder/Decoder
Create an entry for our encoder and decoder in the libavcodec/allcodecs.c like this
…
extern const FFCodec ff_ac3pt_encoder;
extern const FFCodec ff_ac3pt_decoder;
…
Changes in the Makefile
Create an entry for our encoder and decoder in the libavcodec/Makefile like this
…
OBJS-$(CONFIG_AC3PT_DECODER) += ac3ptdec.o
OBJS-$(CONFIG_AC3PT_ENCODER) += ac3ptenc.o
…
Provide Demuxer Connection
Establish a demuxer connection that links the AC3PT codec with the input streams. This step is crucial for ensuring that FFmpeg can properly handle and route AC3 audio data.
Since, we have remapped the AV_CODEC_ID_AC3 to AC3PT enc/dec, we need not to bother about this. However for the ones who have a new codec entirely, need to create a new muxer/demuxer. Read this https://wiki.multimedia.cx/index.php/FFmpeg_demuxer_HOWTO to know more on this area.
Compile and Verify
Our work on the codebase is complete. Now, we need to reconfigure the ffmpeg again by including --enable-encoder=ac3pt and --enable-decoder=ac3pt. Compile with this modified configuration. Successful compilation means that we have successfully enabled AC3PT encoding / decoding in the FFmpeg.
For further details or specific coding examples, you can refer to the FFmpeg codec HOWTO guide here. This resource provides comprehensive instructions and best practices for adding new codecs to FFmpeg.
Limitation of this Method
The downfall in this method is the native lossy AC3 encoding and decoding will not take place atall as the codec AV_CODEC_ID_AC3 is remapped to the newly created AC3PT encoders anddecoders. For the ones who need both, may use their own implementation with certain changes,such as ensuring the codec ID is correctly set in the AVCodecContext and adapting the parsingand buffering logic to accommodate both AC3 and AC3PT formats simultaneously, potentiallythrough conditional checks or separate handling pathways in the code.
Real-World Applications of AC3PT
AC3PT, or AC3 Passthrough, has several real-world applications:
- Home Theater Systems: Many home theater setups utilize AC3PT to deliver high-quality audio from digital media sources to AV receivers without decoding, preserving the original sound quality. This often includes support for Dolby Digital audio.
- Streaming Services: Streaming platforms may use AC3PT to efficiently transmit surround sound audio tracks, ensuring compatibility with various playback devices while maintaining audio fidelity, including Dolby content.
- Broadcast Television: AC3PT is commonly employed in broadcast systems to transmit compressed audio over the air, allowing for better bandwidth management without sacrificing audio quality. This includes Dolby Digital broadcasts.
- Media Players: Software and hardware media players often support AC3PT to enable seamless playback of AC3 and Dolby audio tracks from DVDs or digital files, enhancing the viewer's experience.
- Gaming Consoles: Video game consoles leverage AC3PT for delivering immersive audio experiences, allowing game developers to provide rich soundscapes, including support for Dolby technologies.
- Dolby Atmos Integration: Some advanced audio setups use AC3PT as part of a larger system that includes Dolby Atmos, enhancing spatial audio experiences in both cinema and home environments.
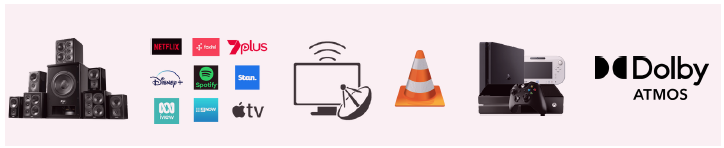
Fig.2 Examples of Real World Applications
Comparative Analysis of AC3 with AC3PT
Let’s have a look at the comparison between AC3 and AC3PT.
Feature | AC3 | AC3PT |
Audio Compression | 1. Uses lossy compression techniques to reduce file size. 2.Supports multi-channel audio (up to 5.1 channels). | 1. Functions as a passthrough; retains the original audio quality without compression. 2. Supports the original AC3 signal. |
Use Case | 1. Commonly used in DVDs, broadcast television, and streaming services. 2. Suitable for applications where file size is a concern. | 1. Ideal for home theaters and professional audio environments. 2. Often used when high fidelity is required, such as in cinema sound systems. |
Latency | 1. Typically has higher latency due to the need for decoding before playback. 2. May introduce delays in realtime applications | 1.Lower latency; audio is sent directly to output without processing. 2.Suitable for real-time applications like gaming or live audio performance. |
File Size Efficiency | 1. Produces smaller file sizes compared to uncompressed formats (like PCM) due to lossy compression. 2. Efficient for storage and streaming bandwidth | 1.Maintains the original bitrate and file size of the audio source. 2. Ideal for scenarios where preserving audio quality is more important than file size. |
Audio Quality | 1. Good quality, but may lose some detail due to compression. 2. Subject to artifacts, especially at lower bitrates. | 1. High fidelity; preserves all nuances of the original audio track. 2. No loss in quality, making it preferred for audiophiles and professionals. |
Support for Dolby Formats | 1. Supports Dolby Digital, but may not support newer formats like Dolby TrueHD or Atmos | 1. Compatible with various Dolby formats; can pass through Dolby Digital and other high-definition audio without loss. |
Conclusion
In conclusion, AC3PT addresses the need for high-quality audio passthrough, offering aneffective solution for maintaining the integrity of AC3 audio streams. Its integration into FFmpegallows developers to leverage advanced audio processing capabilities seamlessly. Real-worldapplications underscore its value in both consumer and professional settings, from streamingplatforms to live events. The comparative analysis reveals how AC3PT enhances workflowsfocused on audio quality, while AC3 remains suitable for efficient compression. Ultimately, thechoice between the two formats should be guided by the specific requirements of a project,enabling content creators to deliver optimal audio experiences. As audio technology advances,AC3PT emerges as a critical asset for those prioritizing quality in their media productions.